This article is for developers, iOS enthusiasts, and anyone who encountered the challenge of ensuring visual coherence in a mobile app.
Let me explain. At times different types of elements in your mobile app have the same look and feel, right? They are coherent. Often, achieving the desired visual consistency, comes at a price: you end up writing many lines of repetitive code, wishing this wouldn’t make everything less maintainable and difficult to change if need be.
One possible solution: constants.
Grouped and used correctly, constants might solve inconsistency issues, but maintainability and code simplicity won’t increase significantly. There must be another way …
While trying to find a simple, elegant solution to the problem, I came up with an interface which defines a single point of entry for customizing the UIKit element throughout the application. The concept is called ViewDecorator.
The core of the pattern is defined with the following protocol, describing how a decorator object should behave:
protocol ViewDecorator {
func decorate(view: UIView)
}
It has a single method, called decorate and takes a single parameter, for the component instance which we intend to decorate.
To make the decoration easier, I created an extension over UIView. With this extension a list of decorators can be passed to the view, without having to call decorate multiple times over it.
extension UIView {
func decorate(_ decorator: ViewDecorator) {
decorator.decorate(view: self)
}
func decorate(_ decorators: [ViewDecorator]) {
decorators.forEach { $0.decorate(view: self) }
}
}
Let’s see this in action.
In the following example a decorator was defined to apply corner radius (CornerRadiusViewDecorator) and another to give a mild drop shadow (ShadowViewDecorator) to our views.
struct CornerRadiusViewDecorator: ViewDecorator {
let cornerRadius: CGFloat
func decorate(view: UIView) {
view.clipsToBounds = true
view.layer.cornerRadius = cornerRadius
}
}
struct ShadowViewDecorator: ViewDecorator {
let radius: CGFloat
func decorate(view: UIView) {
view.layer.shadowRadius = radius
view.layer.shadowOpacity = 0.3
view.layer.shadowColor = UIColor.black.cgColor
view.layer.shadowOffset = CGSize(width: 0, height: radius / 2)
view.layer.masksToBounds = false
}
}
Now let’s say we want to apply both decorators at the same time in our app to achieve a card like appearance. We can use composition and define a new object with the same protocol.
struct CardViewDecorator: ViewDecorator {
private let decorators: [ViewDecorator] = [
CornerRadiusViewDecorator(cornerRadius: 4),
ShadowViewDecorator(radius: 2)
]
func decorate(view: UIView) {
view.decorate(decorators)
}
}
Now, we can use these structs to decorate different kinds of UIKit elements:
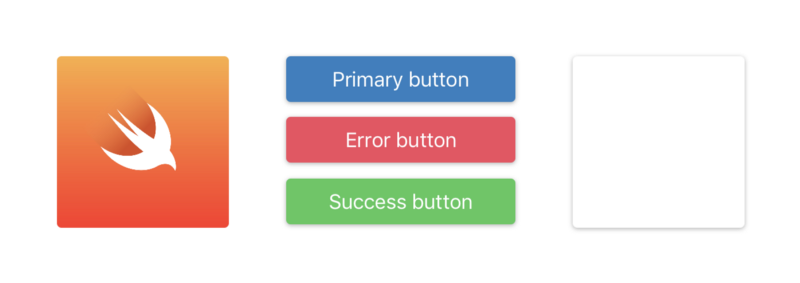
UIImageView without shadow (left), UIButton subclasses (middle), UIView (right)
By keeping the look and feel contained in these separator objects, maintainability and readability increases, the lines of repetitive code decreases. Imagine how easy is to modify the style of your components in case of a rebranding. 😄
Accessing attributes of UIView subclasses
You might face an issue while trying to customize a specific UIView subclass attribute since the protocol takes a specific UIView type.
One possible solution might be to cast the view instance to the expected type inside the decorate(_ view: UIView)
but that would mess up the single responsibility principle and make it behave differently with different kinds of instances.
Associated Types to the rescue!
protocol ViewDecorator {
associatedtype View: UIView
func decorate(view: View)
}
Let’s see how the decorator object changes:
struct ButtonViewDecorator: ViewDecorator {
typealias View = UIButton
func decorate(view: UIButton) {
view.setTitleShadowColor(UIColor.lightGray, for: .normal)
}
}
Quite neat, right?
I’d be happy to hear your opinion about this solution and interesting use cases I might not have thought of.
You can also follow me on Medium.